Node.Js and MySQL
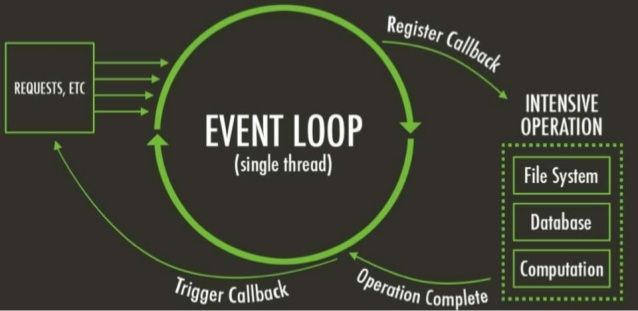
As Week 11 and 12 wrapped up, I am officially half-way through the UT BOOTCAMP. Wow. I can definitely see the growth in my skills and knowledge level. The past two weeks were all about Node.js and MySql. Homework assignments for these two weeks were completed in the terminal and are demonstrated here using tutorial videos. We got a lot to cover, so let's get to learning and discovering.
What is Node.js?
Since Node is such a huge part of Javascript and our class, I feel it is important to dive in a little deeper here.
Node.js is an open source cross-platform runtime environment that is written using Javascript and as defined by it's documentation is:
"A Javascript runtime built on Chrome's V8 Javascript engine"
Let's unpack this really quick:
Javascript Runtime
= the environment that provides built-in libraries available at runtime (or execution time)Javascript Engine
= responsible for producing the machine-executable operations from code written in Javascript
As such Node.js and Chrome share the engine but have different runtime environments.
Since Node.js is cross-platform it can be run on OSX, Microsoft Windows, and Linux (which is a huge plus).
Features
- Asynchronous and Event Driven:
Asynchronous
or non-blocking, meaning that the server doesn't wait for an API to return data before moving on to the next piece of code. Once the API call has finished and is returned, Node.js will then handle it.
- Single-Threaded:
- This means it uses a single thread model with event looping to handle requests. Also because the single-threaded model is non-blocking and can handle many requests at the same time, it makes this platform very scalable opposed to other servers like Apache that create multiple threads to handle requests. The image below captures how Node handles events.
- Speed:
- It is very fast in its code execution since it runs on top of the Chrome Javascript Engine
- No buffering:
- Node.js apps don't buffer any data, they just return them in managable data pieces.
Example of how Node.js uses the event loop:
When to use
- Single Page Applications
- Streaming Applications
- JSON APIs based Applications
- Server-Side Applications
Another great resource for learning Node aside from the documentation mentioned above is here.
Node Package Module and Dependencies
A Node Package Module is basically a packet of pre-built code (modules) that grant the user access to a library of functions or properties that give developers more tools, allow them to build programs faster and give them the ability to interact with other programs like MySql. They are often referred to as dependencies
because if you choose to use say the mysql
package in your project you must require the package withing your javascript file ie: var mysql = require('mysql');
as you are 'dependent' on this module in order for your project to work.
A few helpful links:
One fun fact: Node's package ecosystem is one of the largest open source libraries in the world.
What is MySql?
MySql is an open source relational database built on the SQL architecture. It allows for the storage and retrieval of data. Think of it as a warehouse that stores all of your merchandise and you can send merchandise to your warehouse or retrieve it and send it somewhere else.
There is a lot more to MySql but we will stop here for now. For more in-depth reading the documentation is a great place to start: MySql Documentation
Additionally, here is a great list of shortcut commands for SQL.
Terminal Hangman
Let's see Node.js in action. This hangman takes on the basic hangman rules (similar to the previous hangman I created) except this one cannot be played online, it can only be run on a local terminal.
In order to play on your computer you would have to do the following:
- Download my Github Repo to your computer
- Make your way,
cd
, to the directory you just downloaded - Run
npm install
which will download all of the dependencies used - Run
node main.js
to play
If you are not that ambitious you can see a brief video below to see the result:
Now that you have seen how it functions, how was it built?
The build:
The framework is built in Node.js and I have also used a few npm packages to achieve the look and feel. I have used the npm package Colors which allows the user to call simple .colorName
functions to change the color scheme on your screen. My favorite is .rainbow
which is used in the category description. To make the terminal interface more user-friendly the npm package Inquirer was used.
Bamazon Store
This project displays a table of items that are available to the user to purchase. To build this, the framework used is Node.js and I used the Inquirer npm package again. The main difference here is that I used MySql to handle all of the data. The data that is displayed in the terminal is housed as a table in the database and every time the user purchases an item it decreases the available amount to purchase in the database.
Additionally, to prettify the table output I used the Cli-Table npm package.
Here is a brief overview on how it works:
Until next time... smile and be strong!!
Shout Outs
- Music listened to while blogging: Bill Evans - Days of Wines and Roses
- Node.js Documentation
- MySql Documentation
- NPM Documentation